Creating Command Line Azure DevOps Pull Requests
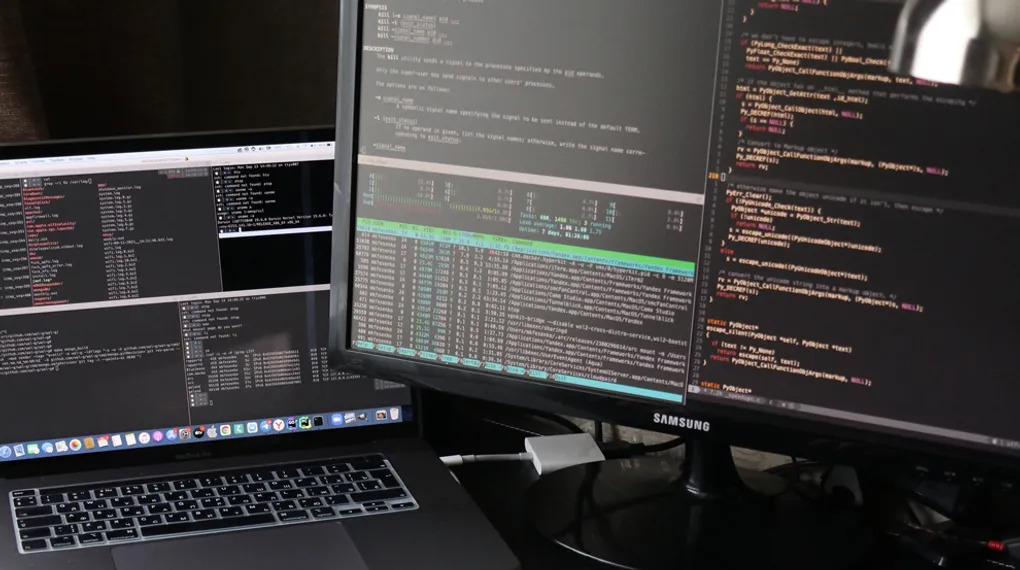
I spend a lot of time on the command line, and I am always looking for ways to improve my productivity. In this post I will share how we can create Azure DevOps pull requests from the command line using the Azure Devops CLI and a little PowerShell.
Az DevOps CLI
Azure DevOps provides an extension to the Azure CLI that allows you to interact with the cloud-based Azure DevOps from the command line. It provides all the functionality you would expect from the Azure DevOps web interface, and just like the Azure CLI is available for Windows, Mac and Linux.
As it is an extension to the Azure CLI, it requires the Azure CLI to be installed first. This extension will then be auto-installed on first use. You can however pre-install the Azure DevOps CLI extension using the following command.
az extension add --name azure-devops
For the rest of this post, we will use the pull request feature of the Azure DevOps CLI however, there are many other features in the getting started guide.
Creating a Pull Request
Creating a pull request using the Azure DevOps Cli is as simple as using the following command.
az repos pr create
The command has 20 different arguments, although none are mandatory as the extension can calculate these from the current git folder and branch. It is rare however, that the calculated values will be exactly as you need so you can see the full details of the optional arguments in the official documentation to customize your pull request.
Automation
The command for creating your pull request with all the arguments you may want can become quite long and requires a lot of information. To make this easier to use we can create a PowerShell function, pre-populate some details and create the pull request for us.
To start with we will open your Powershell profile using this command:
code $profile
This will open the PowerShell profile in vs code, and if the file does not exist it will prompt you to create it. Any functions we add to this can be called at any time by name from your Powershell session.
Once open we can add the following function to the profile.
function PR([String]$title = "") {
#Prompt for Login if needed
$status = (az account show)
if ($status.length -eq 0) {
az login --output none
}
#Calculate the last commit message to use int he PR title
$lastCommitMsg = (git log --pretty=format:"%s" -n 1)
#My branches are named like "feature/1234-branch-name" so we can
#extract the work item id. If no id is found it will default to
#empty and just not link a work item
$wordItemId = ((git branch --show-current) -replace "\D")
#This should get changes since the branch was created
$changes = (git cherry -v main | ForEach-Object{ $_.Split(" ",3)[2] })
#Prompt for Title if not provided
if ($title -eq "") {
$userTitle = Read-Host -Prompt "Enter title or skip to default to '$lastCommitMsg'"
if ($userTitle) {
$title = $userTitle
}
else {
$title = $lastCommitMsg
}
}
#Create PR
Write-Host "Creating Pull Request..."
$result = az repos pr create `
--title $title `
--transition-work-items true `
--auto-complete true `
--delete-source-branch true `
--description $changes `
--work-items $wordItemId `
--detect true `
--open `
--output json | ConvertFrom-Json
$pullRequestId = $result | Select-Object -ExpandProperty pullRequestId
if ($pullRequestId) {
$baseUrl = $result | Select-Object -ExpandProperty repository | Select-Object -ExpandProperty webUrl
write-host "$baseUrl/pullrequest/$pullRequestId"
}
}
This is a long block of code however hopefully you can see what is happening. We use GIT commands and some defaults to create a pull request. We also use the git branch name to extract the work item ID and auto-link the work item to the pull request. It is opinionated to the way I work but should be simple enough to customize for your needs.
Any changes to the Powershell profile will only take place when you create your next Powershell session.
Now this is in place we can create a pull request using the following command.
pr -title "Code Change Pull Request"
Conclusion
In this short post, we looked at the Azure CLI extension for Azure DevOps and how we can use it to create pull requests from the command line. We also looked at how we can automate the process using a Powershell function created in the Powershell profile.
I hope you found this post useful, and if you have any questions or suggestions please leave them below.
Title Photo by Mikhail Fesenko on Unsplash